What is gRPC
gRPC is a networking framework being developed by Google. The gRPC protocol allows for a client to directly call methods on the server. The client and server both implement the gRPC service, and then they are able to communicate directly to each other. gRPC makes use of protocol buffers to handle working with and structuring the data. More information can be found at https://grpc.io/.
Module Setup
The easiest way to setup gRPC is to setup a separate module that can be reference through the Xamarin project. Once this is setup, a protobuf file specific to the server will need to be added to the module. This file will be created as part of the server setup.
1. Create a new folder called Protos in the module and add the .proto file
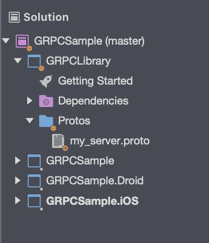
2. Edit the module file and add a reference to the newly added .proto file
<ItemGroup> <Protobuf Include="Protos\my_server.proto" GrpcServices="Client" /> </ItemGroup>
Full file:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.1</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Google.Protobuf" Version="3.12.4" />
<PackageReference Include="Grpc.Tools" Version="2.23.0">
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
<PrivateAssets>all</PrivateAssets>
</PackageReference>
<PackageReference Include="Grpc.Net.Client" Version="2.30.0" />
<PackageReference Include="Grpc" Version="2.30.0" />
<PackageReference Include="Grpc.Net.Common" Version="2.30.0" />
</ItemGroup>
<ItemGroup>
<Folder Include="Protos\" />
</ItemGroup>
<ItemGroup>
<Protobuf Include="Protos\my_server.proto" GrpcServices="Client" />
</ItemGroup>
</Project>
3. Add the following NuGet packages references to the library module. (at the time of this post, the current version is 2.31.0)
- Grpc.Net.Client
- Google.Protobuf
- Grpc.Tools
- Grpc.Core
4. Clean and Build your project
Once these steps are complete, new networking objects will be automatically generated that will be used for making and receiving requests.
Making a request
To make a request, you will first need to create a channel to access the server, and then use the newly created networking object to make a call. When working with gRPC, a channel is a connection that is created which specifies the host and port of the server. It can also include additional data, such as authentication metadata, as shown below.
var credentials = CallCredentials.FromInterceptor((context, metadata) => { metadata.Add("Authorization", "Token”); return Task.CompletedTask; }); var channel = new Channel("myserver.com", 443, ChannelCredentials.Create(new SslCredentials(), credentials)); var client = new MyService.MyServiceClient(channel); var request = new Request(); var reply = client.MakeCall(request);
Android and iOS setup
To work with the gRPC service in Android and iOS, the following NuGet packages will need to be added to both the Android and the iOS modules. This is separate from the step above of adding the NuGet packages to the library module. (Again, at the time of this writing, the version is 2.31.0)
- Google.Protobuf
- Grpc.Core
- Grpc.Tools
- Grpc.Net.Common
- Grpc.Net.Client
- Grpc.Core.Api
Once these items are added, you will be able to interact with the gRPC module created above. Android will be able to make network calls successfully without any additional setup. However, there is a bug with iOS where the newer version of the gRPC libraries do not bind correctly to the Xamarin project. gRPC is technically considered experimental for Xamarin projects, and it does not appear that Google is going to be addressing this problem anytime soon. This bug has been submitted to the gRPC library repo and can be found here: https://github.com/grpc/grpc/issues/19172
For iOS to bind properly, a new xml tag will need to be added to the Grpc.Core.targets file. The file can be found at myProject/packages/Grpc.Core.{Version}/build/Xamarin.iOS10/Grpc.Core.targets. The following tag should be added to both libraries references in that file:
<IsCxx>True</IsCxx>
For details on this, and how to automate this fix, visit: https://techblog.livongo.com/fixing-grpc-xamarin-ios-linking-errors/
Once all of these steps are complete, you will have a newly working Xamarin gRPC client that works for both Android and iOS.